Python Data Types
In programming, the fundamental building blocks of any code are the primitive data types. These little bits of information build…
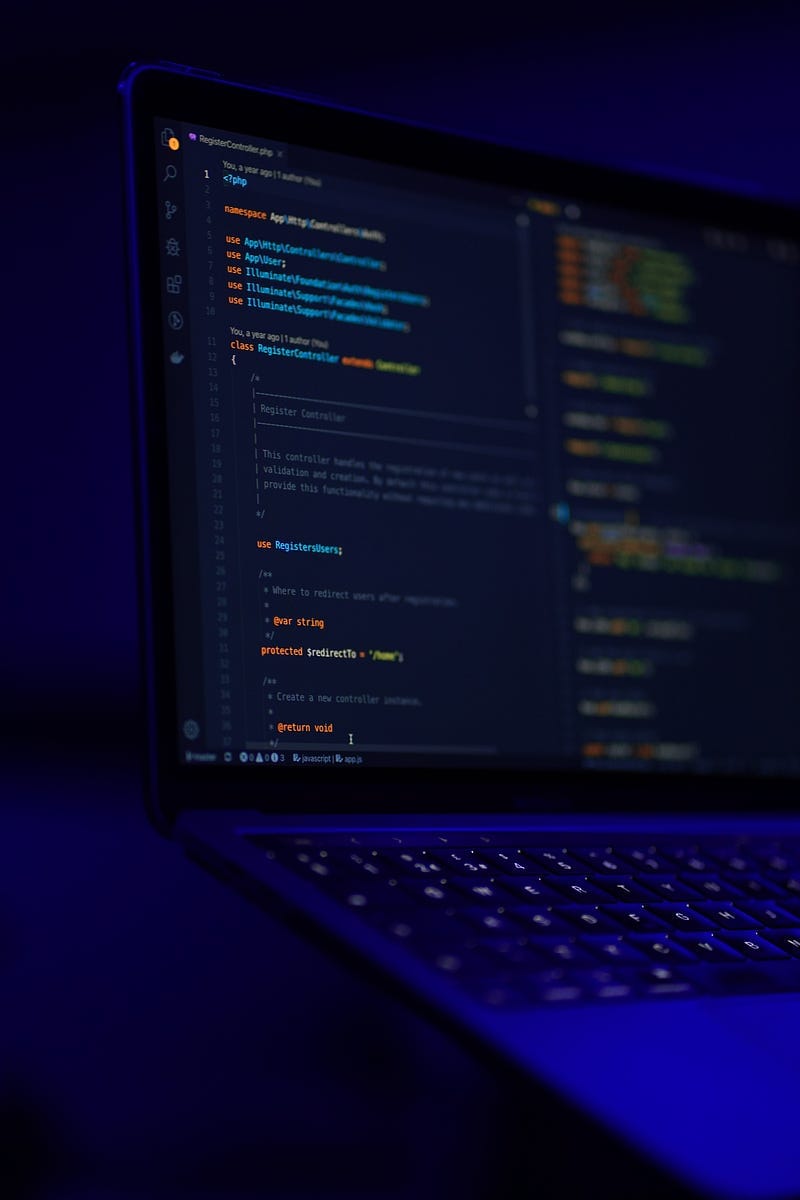
In programming, the fundamental building blocks of any code are the primitive data types. These little bits of information build increasingly complex systems.
What Are Data Types?
Data types are the smallest piece of data that we can use in the context of a Python program. We can combine them in infinite ways to create the programs we want.
Other programming languages have more specific distinctions on the data types, but in Python, we only have to worry about four of them.
- Strings
- Ints
- Floats
- Bools
From these four types of information, we can create anything we can imagine and stand up complex systems. It’s a beautiful thing that lends to Python’s popularity.
Strings
Strings are any sequence of characters surrounded by quotes. In Python, these can be double or single quotes. Even if we have a number inside the quotes, it’s still a string.
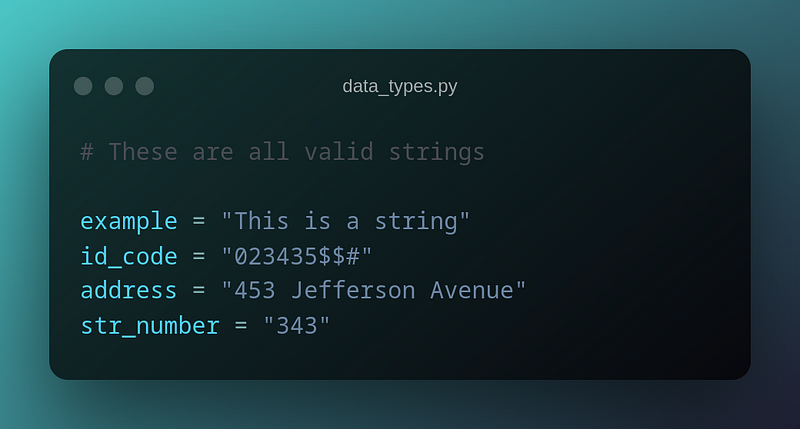
In the context of programming, strings are the most versatile of the data types. We can combine, split, separate, and format them however we like.
We can also multiply and add strings together.
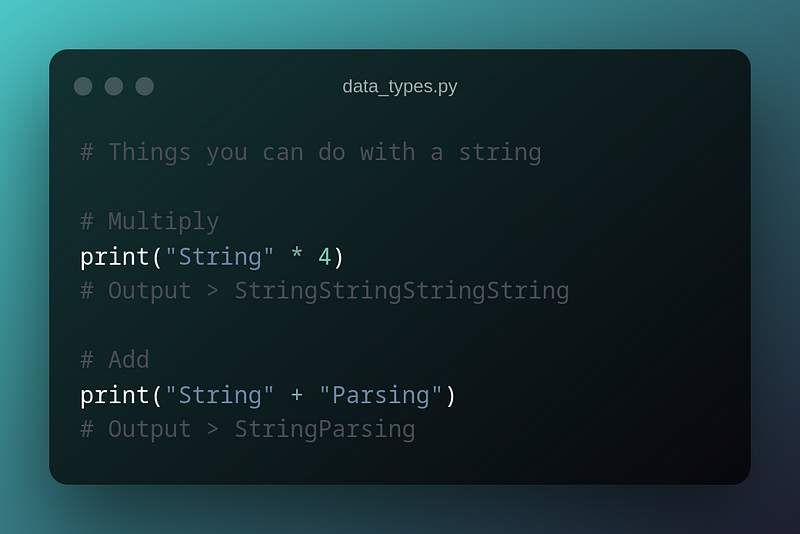
That’s the extent of the mathematical capacity that strings have. While they’re versatile, We can’t do any mathematics with them.
Ints and Floats
Where strings fall short, integers and floating point numbers fill the gap. These data types exist to serve one purpose, math. Python knows how to perform calculations with them as we would expect.
- Add
- Subtract
- Multiply
- Divide
- Modulus division — Getting the remainder
- Integer division — Forcing a whole number
The primary difference between floating point numbers (float) and integers (int) is that a float uses a decimal (floating) point while the int is a whole number. Otherwise, they behave almost the same way.
One caveat to pay attention to is that in Python, these two data types can change over time. If we combine an int with a float, we will get a float. If we combine a float with a float, we will get a float. Additionally, if we divide an int by an int, we will also get a float. This is due to the way Python handles the behavior of data types. They’re not locked, The official term is the Dynamic Type System.
Booleans
This data type is notorious for confusing beginners. Booleans (bool) are nothing more than a switch. It can either be on or off.
It is useful for us programmers because sometimes we need a specific condition to be met, or to toggle a specific sequence of code. It’s a universal Yes or No system baked into all programming languages.
They are especially useful when we use if statements.
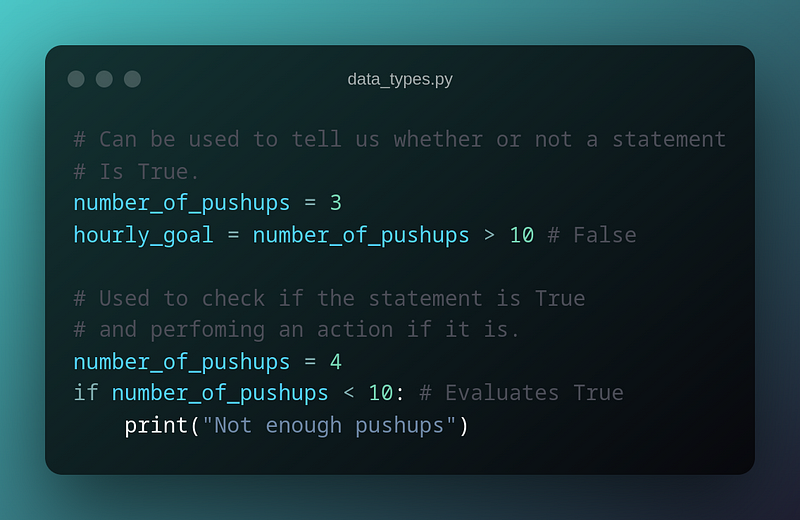
Converting Data Types
Since not all data types work in the same ways, sometimes we want them to be different. In Python, we have the power to convert one data type to another. The official term for this is called Type Casting. Python makes this very easy for us.
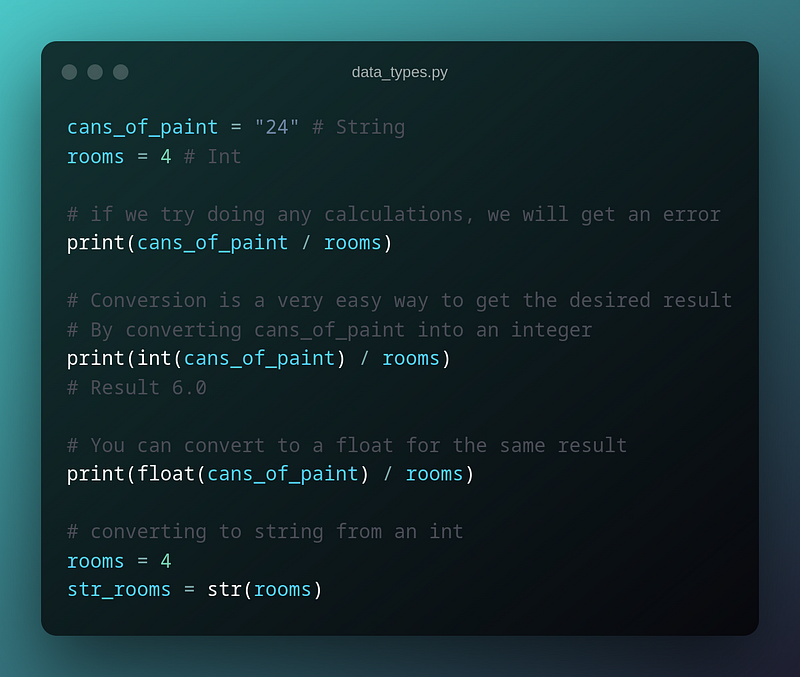
Learning the behavior
In the future, when creating more complex combinations of code such as functions, knowing the data types well will make you a much more competent programmer. Once the fundamentals are there, they will stay there. I recommend jumping into your editor of choice and learning the behavior, especially for a language like Python.